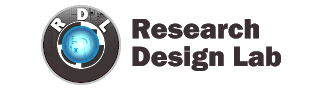
Program:
/* Connections *//* Switch = P2.5
* led = P2.0
* buzzer = P2.1
* relay = P2.2
*/
#include <lpc17xx.h>
#include "blink.h"
#define Switch 5
#define Led 0
#define buzzer 1
#define relay 2
/* start the main program */
int main()
{
uint32_t switchStatus;
/* Initialize the output pins for led, buzzer and relay */
GPIO_Init_Output(buzzer);
GPIO_Init_Output(Led);
GPIO_Init_Output(relay);
/* Initialize the input pin */
GPIO_Init_Input(Switch);
LED_OFF(Led);
LED_OFF(buzzer);
LED_OFF(relay);
while(1)
{
/* Turn On all the leds and wait for one second */
switchStatus = GPIO_Read(Switch); /* Read the switch status */
if(switchStatus == 1) /* Turn ON/OFF LEDs depending on switch status */
{
LED_ON(Led);
LED_ON(buzzer);
LED_ON(relay);
}
else
{
LED_OFF(Led);
LED_OFF(buzzer);
LED_OFF(relay);
}
}
}
Hardware Connection
Connect P2.5 to switch (development board)
Connect P2.0 to led (development board)
Connect P2.0 to led (development board)
Connect P2.0 to led (development board)
Output
When the switch is pressed LED is ON, buzzer is ON and relay is ON.
When the switch is not pressed LED is OFF, buzzer is OFF and relay is OFF.
FOR DETAIL INFORMATION ABOUT ARM CORTEX DEVELOPMENT KIT : CLICK HERE